Ignore this intro
Hello blog. Hi Jimmy, said the blog. Wait…
Because I really hate reading boring articles and get distracted easily, I’m going to try and make all my posts as interesting as possible, and coenside this with the comments in my code.
I know a guy called Coen actually.
Organising the assignment
The first thing that needs to happen is getting a Visual Studio solution set up to run this game. This is probably the most fun part because it;s easy and you can’t cause bugs that take hours to complete.
Wrong. I have to do planning first.
- Come up with game idea
- Do planning and UMLs
- Prepare solution
- Write main code and get engine running without errors
- Create classes for objects and nodes etc.
- Create terrain using classes and generation
- Add lava and lava effects
- Add textures
- Add particles
- Add fine details like fog, extra shadows
- Tweaking things so they look special (the good kind)
My understanding of this ass iron mint
Basically make a demo scene with a camera that I can control to show it the F off.
It has to have:
- A light
- A camera
- An FBX model
- A shadow
- Generated terrain
- Particles
- Basic scene graph
- Everything textured and lit with lights properly
What I do
I’m planning on making a volcano scene. I was and still am inspired by the volcano level (Eridium Blight) in Borderlands 2. Here’s some pictures:


I sat there in Borderlands 2 for probably 30 minutes looking at the detail that went into this lava and I noticed the following:
- The lava has a layer underneith that has a water/blur effect on it
- There’s soot floating through the air that lands on your face (like snowflakes)
- There’s smoke coming out of the lava
- There’s heat waves
- The lava texture flows in one direction rather than just sits there
- The lava has a glow effect on it
- There’s hot looking particles bubbling out of the lava
- The lava mesh is slightly wavey and not just static
This is a lot better than the deadful lava in Starcraft II:

OH GOD IT BURNS MY EYES. Not in a lava burn way, but a “I can’t stand to even look at it” way. It’s low resolution, doesn’t animate, and looks flat. It doesn’t even appear like it’s bright. It looks more like a Persian rug is coming up to destroy all my units and buildings.
My scene won’t actually have an erupting volcano, but I do want some particles coming out of it like black smoke, soot and bubbles of lava. Also snow-like soot like on Borderlands.
I now have the lava down pat. The next thing is the scene generation.
I plan on having a hight map generation using perlin noise, over the top of another map that contains the volcano’s “norm” shape. Something similar to the following:

Combined would look something like this:
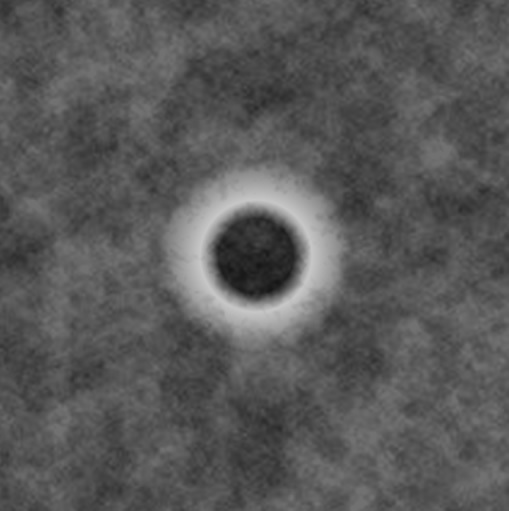
Then, I’d add a plane of lava just around the lowest Y area and make sure the 2 hight maps together don’t go too low. You will notice that the centre of the volcano ring overlay is darker than the outside. This is the key to having the lava only in the volcano. It might even have a few rocks pointing out of the lava, which would be okay.
Beautiful.
I don’t plan on generating the volcano ring (at this state, although it’d be possible because I just made it with an oval gradiant in Fireworks) but If I just use the image I could play around with the shape of the volcano, maybe give it some natural looking veins or something.
Lights and stuff
The light source will be a skylight coming from the sky, and an orange one coming from the volcano. This will ensure the inside of the volcano’s walls are lit up by the lava. There will also be a general diffuse colour around the rocks.
Because I want to do shadow maps, I’ll be adding in blurred shadows. This is because The scene is going to be cloudy.
FBX models
I’ve combed TurboSquid.com for trees. I thought that I could randomly place a set number of dead trees around the map (avoiding the volcano) using circle maths for distance. These should be textured, lit and cast shadows. Naturally.
Advanced features
For my advanced bit, I’m adding in advanced shadows because shadows are kick-ass like unicorns. So the light source is going to be a sun. Again, the scene is dark and cloudy, so I want to try and blur the shadows a bit to appear like ones in a cloudy-ish sky.
Other notes
The camera will just be a normal one much like the AIE Framework camera. WASD will move it, and the mouse will aim it. Nothing too fancy.
The scene will be set up using a scene graph. This will be represented by simply having all the trees as children to the landscape. It won’t be dramatically noticable but it will be made like that.
I’ll add a transparent, cloudy plane as the sky. If I have time (and can figure it out), I’ll add a skybox.
There will be a sort of fog in the game. This will make the landscape nicer and hopefully cut off the edges of the map so you can’t see the box where the hills are. The only issue with the fog would be the lighter parts of the map should shine through more than the darker ones. This will be a challenge. For example, looking at the sun through fog.